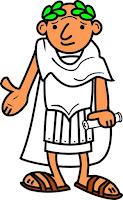 |
Caesar Cipher |
Caesar Cipher is one the most simplest encryption techniques. It can be easily be decrypted using frequency counts. This program uses this simplest of the decryption techniques so is not likely to be the best but is definitely the easiest to code and understand. To understand how this program works you must have a thorough knowledge of ASCII characters.
Links:-
Program-Caesar Cipher Encryption cum Decryption
- Language:-C
- Tested on:- Linux x86 (Compile and use it is likely to work on both windows and linux).
- Author:-Abhishek Munagekar
- This program requires two text files input and the output file.
- The input file is input.txt and it must be created with the input text already in it whether it is for encryption or decryption.It must be present in the same file as the executable.
- The output file will be output.txt. This may or may not be present. The program will create one if there isn't one else it will overwrite the existing file.
- Please test this program on English articles and not on program codes, other languages. Random text seems to work fine. Generate random text from the link specified or you can use any English newspaper article , magazine content etc.
- Note:-Do not use this for encrypting any important information as this cipher can be cracked in seconds.
- Possible Applications:-
- Strengthen your password:- One of the most common passwords used is the word password itself. This is one of the first tries for anyone trying to crack your password. Consider this password->sdvvzrug when shifted by 3. This word is unlikely to be tried by any hacker as his first try.
Code
/*
============================================================================
Name : Caesar.c
Author : Abhishek Munagekar
Version :1.0
Website : Visit www.facebook.com/prgwonders and www.prgwonders.blogspot.in
Description : Caesar Cipher Encryption cum Decryption
============================================================================
*/
#include < stdio.h >
#include < stdlib.h >
void enc(int shift) {
char c;
FILE * in , * out; in = fopen("input.txt", "r");
out = fopen("output.txt", "w");
while ((c = fgetc( in )) != EOF) {
if (c >= 65 && c <= 90) { //if lowercase
c = c + shift;
if (c > 90)
c = c - 26;
fputc(c, out);
} else if (c >= 97 && c <= 122) { //if uppercase
c = c - 26 + shift;
if (c < 97)
c = c + 26;
fputc(c, out);
}
}
fclose( in );
fclose(out);
puts("Caesar cipher encryption is complete");
}
void dec() {
float temp;
int temp2;
float latency;
int i, j, shift, flag = 0;
//calarray contains standard english letter frequency counts
float calarray[26] = {
8.167,
1.492,
2.782,
4.253,
12.702,
2.228,
2.015,
6.094,
0.153,
0.772,
4.025,
2.406,
6.749,
7.507,
1.929,
0.095,
5.987,
6.327,
9.056,
2.758,
0.978,
2.361,
0.150,
1.974,
0.074
};
int farray[26]; //will contain actual frequency count
int chararray[128];
for (i = 65; i <= 90; i++) {
chararray[i] = 0;
}
for (i = 97; i <= 122; i++) {
chararray[i] = 0;
}
FILE * in ;
float count = 0;
char c = 'a'; in = fopen("input.txt", "r");
while ((c = fgetc( in )) != EOF) {
if ((c >= 65 && c <= 90) || (c >= 97 && c <= 122)) {
chararray[(int) c]++;
count++;
}
}
count = count / 100;
for (i = 0; i <= 25; i++) {
farray[i] = chararray[i + 65] + chararray[i + 97]; //actual frequency count
calarray[i] = calarray[i] * count; //expected frequency count
}
puts("Printing latency table");
for (j = 0; j <= 25; j++) { //iterate for all shifts
latency = 0;
for (i = 0; i <= 25; i++) { //iterate over each letter
temp2 = i - j;
if (temp2 < 0)
temp2 = temp2 + 26;
temp = farray[temp2] - calarray[i]; //find the difference
if (temp > 0)
latency += temp;
else
latency -= temp;
}
printf("\nshift %d=%d ", j, (int) latency);
}
puts("\nEnter the value for the shift which has the lowest latency");
puts("If u do not find this correct try for the next lowest and so on");
while (flag == 0) {
puts("Please enter the shift value");
scanf("%d", & shift);
enc(shift);
puts("Shifting operation has been completed. Check if output is correct");
puts("if output is correct press 1 else press 0");
scanf("%d", & flag);
}
}
int main(void) {
int choice;
puts("Caesar Cipher Project"); /* Intro */
puts("Program by Abhishek Munagekar");
puts("For more codes visit www.facebook.com/prgwonders
or www.prgwonders.blogspot.in");
puts("Ensure that u have content in input file and output file.");
puts("input.txt should contain the input to the program");
puts("Enter your choice");
puts("1.Encryption 2.Decryption");
scanf("%d", & choice);
if (choice == 1) {
int shift;
puts("Enter the value for the shift parameter");
scanf("%d", & shift);
enc(shift);
} else if (choice == 2) {
dec();
} else {
printf("\nInvalid choice entered");
printf("\nProgram terminated");
}
puts("Program by Abhishek Munagekar");
puts("For more codes visit our website Programming Wonders:-
www.prgwonders.blogspot.in");
puts("or visit www.facebook.com/prgwonders");
puts("Program Terminated");
return EXIT_SUCCESS;
}
No comments:
Post a Comment