Q: Write an ALP (Assembly Language Program) to find the average of hexadecimal numbers stored in an array.
Assembler:- NASM
There are two parts:-
This conversion routine is explained through comments in the code
student@pc:~$ ld -m elf_i386 -o avg avg.o
student@pc:~$ ./avg
0Dstudent@pc:~$
Software & Hardware Used
OS : Linux - 64 (Ubuntu 14.04 LTS)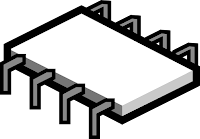
There are two parts:-
- Finding the average of the hex numbers :
- Converting the obtained average to ASCII
Average
The average is found out by finding the sum of all the numbers and dividing it by the total number of numbersASCII Conversion
Refer the ASCII chart. You will find that two types of conversion formula are present while converting a symbols which refer the hexadecimal numbers into ASCII.Case 1: 0 to 9
In this case we simply add 30H( ie.., 48 in decimal )Case 2: A to F
In this case we need to add 37H(ie..,55 in decimal).This conversion routine is explained through comments in the code
Code
macro disp 2
mov eax,04H
mov ebx,01H
mov ecx,%1
mov edx,%2
int 80H
%endmacro
section .data
msg1: db ""
msg2: db ""
array : db 01h,15h,15h,06h,28h,03h,02h,06h,05h,19h
section .bss
count: resb 01
tot : resb 01
answer : resb 02
global _start
section .txt
_start:
mov byte[count] ,10
mov esi ,array
mov al,byte[esi]
mov [tot],al
begin:
inc esi
mov al,byte[esi]
add byte[tot],al
dec byte[count]
jnz begin
mov ah,0h
mov al,[tot]
mov bl,0Ah
div bl
mov esi,answer
call convert
disp answer,2
mov eax,01 ;exit system call
mov ebx,00
int 80H
convert: ;this is the function to convert the hex value to ascii
mov byte[count],02 ;initialize the counter
l: rol al, 04H ;Moving MSD to LSD
mov bl, al
and bl, 0FH ;Masking the higher bits
cmp bl, 09 ;Compare with nine
JBE m ;If below or equal jump to label m
add bl, 07H ;else add correction factor
m: add bl, 30H
mov [esi], bl ;store ASCII value in esi
inc esi
Dec byte[count]
JNZ l
RET
Output
student@pc:~nasm -f elf avg.asm.ostudent@pc:~$ ld -m elf_i386 -o avg avg.o
student@pc:~$ ./avg
0Dstudent@pc:~$
No comments:
Post a Comment