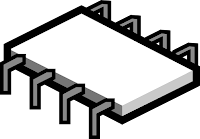
Q:- Write a 64 bit ALP to perform String Operations. The program should be menu driven with the following options:-
- Length of String
- Reverse the String
- Palindrome Test
Code
- Assembler:-NASM
- OS :- Fedora(Linux 64 bit)
- Debugger:-GDB
;Author:-Abhishek Munagekar
;Exp:- String Operations
;macros begin
%macro write 2 ;will be used to write on the screen
mov rax,01h
mov rdi,0h
mov rsi,%1
mov rdx,%2
syscall
%endmacro
%macro read 2 ;will be used to read from the screen
mov rax,0h
mov rdi,1h
mov rsi,%1
mov rdx,%2
syscall
%endmacro
;macros end
section .data
msg1: db 10,"==========================",10
db " STRING OPERATIONS ",10
db "==========================",10
db "Enter your choice",10
db "1.Calculate the length of string",10
db "2.Reverse the string ",10
db "3.Palindrome test",10
db "4.Exit",10
len1: equ $-msg1
msg2: db "Enter the string on which the operation is to be performed",10
len2: equ $-msg2
msg3 : db "The length of the string is "
len3: equ $-msg3
msg4: db "Yes , it's a palindrome",10
len4: equ $-msg4
msg5: db "No, it's not a palindrome",10
len5: equ $-msg5
section .bss
orgstr resb 50 ;original string
revstr resb 50 ;reverse string
choice resb 2 ;to accept the choice
wordlen resb 1 ;length of the string
ascii resb 2 ;len in ascii
count resb 1
section .text
global _start:
_start:
write msg1,len1 ;display menu
read choice,2
cmp byte[choice],31h
je length
cmp byte[choice],32h
je rev
cmp byte[choice],33h
je pal
cmp byte[choice],34h
je exit
jmp _start ;code will reach here if invalid choice is entered
rev: ;the reversal option
mov eax,0
write msg2,len2
read orgstr,50
dec al
mov byte[wordlen],al
mov byte[count],al
mov esi,orgstr
mov edi,revstr
add esi,eax
dec esi ;esi at the end of the orgstr
call thereverser
write revstr,50
jmp _start
length: ;the length option
write msg2,len2
mov al,0 ;initialize al with zero
read orgstr,50
mov byte[wordlen],al
dec byte[wordlen] ;now len has the length of the string without the null character
mov esi,wordlen
mov edi,ascii
call h2a ;function to convert hex to ascii
write ascii,2
jmp _start
pal: ;the palindrome checking option was selected
mov eax,0
write msg2,len2
read orgstr,50
dec al
mov byte[wordlen],al
mov byte[count],al
mov esi,orgstr
mov edi,revstr
add esi,eax
dec esi ;esi at the end of the orgstr
call thereverser
mov esi,orgstr
mov edi,revstr
mov byte[choice],0 ;now choice is used as a flag
loop3:
mov al,byte[edi]
cmp al,byte[esi]
je noflag
mov byte[choice],1
noflag:
dec byte[count]
jnz loop3
cmp byte[choice],1
je nopal
write msg4,len4
jmp _start
nopal:
write msg5,len5
jmp _start
;============================================================
exit: ;to exit from the program
mov rax,60
mov rdx,0h
syscall
h2a: ;function for conversion from hex to ascii
mov byte[count],2
mov al,byte[esi]
loop:
rol al,04
mov bl,al
and bl,0Fh
cmp bl,09h
jbe nocorrection
add bl,7h
nocorrection:
add bl,30h
mov byte[edi],bl
inc edi
dec byte[count]
jnz loop
ret
thereverser: ;esi@end of orgstr ,edi@revstr
loop2:
mov al,byte[esi]
mov byte[edi],al ;moving character by character
inc edi
dec esi
dec byte[count]
jnz loop2
mov byte[edi],10
ret
Ouput
[secomp@localhost asgn4]$ nasm -f elf64 string.asm
[secomp@localhost asgn4]$ ld -o string string.o
[secomp@localhost asgn4]$ ./string
==========================
STRING OPERATIONS
==========================
Enter your choice
1.Calculate the length of string
2.Reverse the string
3.Palindrome test
4.Exit
1
Enter the string on which the operation is to be performed
small
05
==========================
STRING OPERATIONS
==========================
Enter your choice
1.Calculate the length of string
2.Reverse the string
3.Palindrome test
4.Exit
1
Enter the string on which the operation is to be performed
this is a very big string
19
==========================
STRING OPERATIONS
==========================
Enter your choice
1.Calculate the length of string
2.Reverse the string
3.Palindrome test
4.Exit
2
Enter the string on which the operation is to be performed
name
eman
==========================
STRING OPERATIONS
==========================
Enter your choice
1.Calculate the length of string
2.Reverse the string
3.Palindrome test
4.Exit
3
Enter the string on which the operation is to be performed
name
No, it's not a palindrome
==========================
STRING OPERATIONS
==========================
Enter your choice
1.Calculate the length of string
2.Reverse the string
3.Palindrome test
4.Exit
3
Enter the string on which the operation is to be performed
madam
Yes , it's a palindrome
==========================
STRING OPERATIONS
==========================
Enter your choice
1.Calculate the length of string
2.Reverse the string
3.Palindrome test
4.Exit
4
[secomp@localhost asgn4]$
No comments:
Post a Comment