Q: Write a ALP to multiply two 8 bit numbers using Shift-Add Method and Repeated Addition Method. Accept the numbers from the user. Make the program a menu driven one.
Assembler:-NASM
OS:- Linux-64 bit(Fedora)
[secomp@localhost as6]$ ld -o as6 as6.o
[secomp@localhost as6]$ ./as6
=========================================
MENU
=========================================
1.Successive Addition
2.Shift and Add Method
3.Exit
1
Enter the value for the first number multiplicand
09
Enter the value for the second number multiplier
11
0099
=========================================
MENU
=========================================
1.Successive Addition
2.Shift and Add Method
3.Exit
2
Enter the value for the first number multiplicand
32
Enter the value for the second number multiplier
10
0320
=========================================
MENU
=========================================
1.Successive Addition
2.Shift and Add Method
3.Exit
3
[secomp@localhost as6]$
Code:
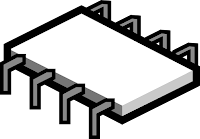
OS:- Linux-64 bit(Fedora)
;Author:-Abhishek Munagekar ;Expno:- 6 ;Title:- Multiplication of 8 bit values ;macros begins %macro read 2 mov rax,00h mov rdi,01h mov rsi,%1 mov rdx,%2 syscall %endmacro %macro write 2 mov rax,01h mov rdi,00h mov rsi,%1 mov rdx,%2 syscall %endmacro ;macros end section .data msg1: db 10,"=========================================",10 db " MENU ",10 db "=========================================",10 db "1.Successive Addition",10 db "2.Shift and Add Method",10 db "3.Exit",10 len1: equ $-msg1 msg2: db "Enter the value for the first number multiplicand",10 len2: equ $-msg2 msg3: db "Enter the value for the second number multiplier",10 len3: equ $-msg3 section .bss count resb 1 choice resb 2 amultiplier resb 3 ;ascii amultiplicand resb 3 ;ascii multiplier resb 1 ;hex multiplicand resb 2 ;hex,extra for shifting answer resb 2 ;hex aanswer resb 4 ;ascii section .text global _start: _start: write msg1,len1 read choice,2 cmp byte[choice],31h je sucadd cmp byte[choice],32h je shadd cmp byte[choice],33h je exiter jmp _start ;jump to start if invalid choice sucadd: ;code for successive addtion here write msg2,len2 read amultiplicand,3 mov r8,amultiplicand mov r9,multiplicand mov byte[count],2 call a2h write msg3,len3 read amultiplier,3 mov r8,amultiplier mov r9,multiplier mov byte[count],2 call a2h ;now we have hex value in place mov word[answer],0h movsx r10,byte[multiplicand] loop2: add word[answer],r10w dec byte[multiplier] jnz loop2 ;now we have the answer at the memory location answer call h2a write aanswer,4 jmp _start ;====================================================== shadd: ;code for shift and add here write msg2,len2 read amultiplicand,3 mov r8,amultiplicand mov r9,multiplicand mov byte[count],2 call a2h write msg3,len3 read amultiplier,3 mov r8,amultiplier mov r9,multiplier mov byte[count],2 call a2h ;now we have the hex values in place mov byte[count],8 mov word[answer],0h movsx ax,byte[multiplier] mov bx,word[multiplicand] mov cx,0 ;cx will store the sum of the partial products shaddloop: rcr ax,01 jnc nocarry add cx,bx ;add if carry nocarry: shl bx,01 dec byte[count] jnz shaddloop mov word[answer],cx ;answer obtained call h2a write aanswer,4 jmp _start exiter: mov rax,60 ;exit system call mov rdx,01h syscall a2h: ;ascii to hex r8 source ,r9 destination, counter at count mov bl,0h loop: rol bl,4 mov al,byte[r8] sub al,30h cmp al,9h jbe nocorrection sub al,7h nocorrection: add bl,al inc r8 dec byte[count] jnz loop mov byte[r9],bl ret h2a: ;r9 destination,counter is set to 4,source is set to answer mov r9,aanswer mov byte[count],4 mov bx,word[answer] loop3: rol bx,04 mov al,bl and al,0Fh cmp al,9 jbe def add al,7h def: add al,30h mov byte[r9],al inc r9 dec byte[count] jnz loop3 ret ;========================================
Output
[secomp@localhost as6]$ nasm -f elf64 as6.asm[secomp@localhost as6]$ ld -o as6 as6.o
[secomp@localhost as6]$ ./as6
=========================================
MENU
=========================================
1.Successive Addition
2.Shift and Add Method
3.Exit
1
Enter the value for the first number multiplicand
09
Enter the value for the second number multiplier
11
0099
=========================================
MENU
=========================================
1.Successive Addition
2.Shift and Add Method
3.Exit
2
Enter the value for the first number multiplicand
32
Enter the value for the second number multiplier
10
0320
=========================================
MENU
=========================================
1.Successive Addition
2.Shift and Add Method
3.Exit
3
[secomp@localhost as6]$
No comments:
Post a Comment