![]() |
Calendar |
How to create a Calendar ?
Creating a calendar is quite easy. Consider the following facts first.- A normal year has 365 days.
- A leap year has 366 days.
- Also 365%7=1 while 366%7==2
- So normally if January1 is a Monday for a year x for the next year(x+1) January 1 will be Tuesday. However if year x is a leap year then for the next year January 1 will be a Wednesday.
1>Finding the day for the Jan 1 of any year.
2>Finding the day for First of the Month for this year.Use a leap correction function so as to correct the first of the month day.(In leap year after feb the day needs to be shifted by one again).
3>Decide on how u wish to display the data.And write Graphics accordingly.
This calendar will work for years greater than 1600. If u wish to find for days less than the year 1601 the diff variable will become negative. Then modulus will become complicated and logic will become hard to understand.
Solution-Part 1 Jan1
- We firstly need to select a base year for which we know the day for Jan1.
- Lets select 1600 as base year.
- Assign values 0 for Monday, 1 for Tuesday and so on. Call this as the day value.
- Lets consider User gives input year =yearx.
- Let diff=(yearx-1600)%400(The calendar repeats after every 400 years)
- day=(diff+day)%7 (We are only interested in finding the day).
- Now we need to find the correction for the leap years
- leap=diff/4
- leap100=diff/100
- leapcorrection=leap-leap100
- day=day+leapcorrection+1(since 1600 is leap year)
- day=day%7
- This gives day for Jan1 of that year.
- This is pretty simple. Just find out the difference between the first of the month and Jan1.And find difference%7.Add this difference to the day value that was previously calculated and perform a %7 operation.
- For leap year add an extra day if month is after Feb.
- This will give you the Month first day.
- Just remember how many days are to be displayed.
- February is a tricky case
- Decide on the interface to be used.(I have used text based graphics, this was one of my earliest programs ,:-) ).
Code
- This code and compiled .exe file works perfectly on Windows 32 bit Operating System. For 64 bit u might have to recompile the file or try compability mode.
- This code uses text based Graphics. The weird numbers in the code are ascii value for characters.
- This code works with directional keys. Directional keys can be used to change the year or month and update the display immediately.
- You can also download this code in .c format along with compiled .exe file at the following mediafire link:-http://www.mediafire.com/?g71862zc76c0w
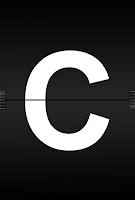
#include<stdio.h> #include<conio.h> #include<string.h> int getkey();void fmonthname(int m); char *monthname[12]; int day=0,leap,fstart,fend; void main() { int scankey,year,month,jan1=0,i,j,k; int montharray[42]; clrscr(); puts("Calendar_v1.0-The portable lite .exe calendar"); puts("A prg by Abhishek Munagekar"); puts("This is a free version and supports 1700-8000 yrs only."); puts("For bug reports contact avm.abhishek@gmail.com"); puts("Press any key to continue"); scankey=getkey(); clrscr(); yearmonth: puts("type the year and month as shown below"); puts("yyyy<space>mm"); scanf("%d%d",&year,&month); clrscr(); actualc: if(year<1700||year>8000||month<1||month>12) { printf("Syntax error, fatal error re-enter all values"); goto yearmonth; } /* core function begins*/ for(i=0;i<=41;i++) montharray[i]=0; /*leap chkr begins*/ leap=0; if((year%4)==0) leap=1; if((year%100)==0) leap=0; if((year%400)==0) leap=1; /*leap chkr ends*/ /*sets month name and day count*/ day=0; fmonthname(month); /*sets month name and day count above*/ /*JAn1day seterbelow*/ jan1=0; jan1=((year-1601)+((year-1601)/4)-((year-1601) /100)+((year-1601)/400))%7; /*leap corrector below*/ if(leap==1&&month>2) day=day+1; /*final start day decider below*/ fstart=(day+jan1)%7; /*array data feeder starts*/ for(i=0;i<fend;i++) { montharray[i+fstart]=i+1; } /*array data feeder ends*/ /*basic info display below*/ printf("\nPrg has captured the following month:-\n"); printf("%d%d/%d\n",month/10,month%10,year); printf("%c%c%c%c%c%c%c\n",196,196,255,196,196, 196,196); printf("Displaying %s,%d\n",*monthname,year); /*display of calendar begins-1st line below*/ printf("\n%c",218); for(i=1;i<=20;i++) printf("%c",196); printf("%c",191); /*2 line now*/ printf("\n%cMo Tu Wd Th Fr Sa Su%c",179,179); /*core display lines now*/ j=0; for(i=1;i<=6;i++) { printf("\n%c",179); for(k=1;k<=6;k++) { if(montharray[j]==0) printf("%c%c%c",255,255,255); else printf("%d%d%c",montharray[j]/10,montharray[j] %10,255); j=j+1; } if(montharray[j]!=0) printf("%d%d%c",montharray[j]/10,montharray[j] %10,179); else printf("%c%c%c",255,255,179); j=j+1; } /*finish display lines below*/ printf("\n%c",192); for(i=1;i<=20;i++) printf("%c",196); printf("%c\n",217); /*display of calendar ends*/ /*new control structure begins*/ puts("\npress any control 2 continue"); puts("escape key :- termination"); puts("left/right arrow key:- previous/next month"); puts("up/down arrow key:- next/previous yr same month"); puts("D letter:- To renter date"); scankey=getkey(); if(scankey==1) { clrscr(); printf("Termination sequence requested by user"); goto terminator; } else if(scankey==75) { clrscr(); month=month-1; if(month==0) { month=12; year=year-1; } goto actualc; } else if(scankey==77) { clrscr(); if(month==12) { month=1; year=year+1; } else month=month+1; goto actualc; } else if(scankey==72) { clrscr(); year=year+1; goto actualc; } else if(scankey==80) { clrscr(); year=year-1; goto actualc; } else if(scankey==32) { clrscr(); printf("\nDirecting to reeenter values..."); goto yearmonth; } else { clrscr(); goto actualc; } /*terminator*/ terminator: puts("Hope u liked the program"); puts("For bug reports mail me:-avm.abhishek@gmail.com"); getch(); } #include"dos.h" getkey() { union REGS i,o; while(!kbhit()) ; i.h.ah=0; int86 (22,&i,&o); return(o.h.ah); } void fmonthname(int m) { switch (m) { /*switch bracket above*/ case 1: *monthname="January"; fend=31; break; case 2: *monthname="February"; day=day+31; fend=28; if(leap==1) fend=29; break; case 3: *monthname="March"; day=day+59; fend=31; break; case 4: *monthname="April"; day=day+90; fend=30; break; case 5: *monthname="May"; day=day+120; fend=31; break; case 6: *monthname="June"; day=day+151; fend=30; break; case 7: *monthname="July"; day=day+181; fend=31; break; case 8: *monthname="August"; day=day+212; fend=31; break; case 9: *monthname="September"; day=day+243; fend=30; break; case 10: *monthname="October"; day=day+273; fend=31; break; case 11: *monthname="November"; day=day+304; fend=30; break; case 12: *monthname="December"; day=day+334; fend=31; break; } /*month name is now set*/ } /*fmonthname function ends here*/
No comments:
Post a Comment